M-Files VaultMap
M-Files.VaultMap is object mapper for .NET, that can be used by any library that works with data of M-Files vault. It supports read, write, delete and search of m-files vault data, through mapped vault-class entities. Best comparison of it can be made with "Entity Framework - Code First to existing database".
Get started
To better understand how VaultMap can be used, we prepared an example with step-by-step walkthrough that will introduce you with basic principles. Whole example can be downloaded here, and you will also have possibility to download it in separate pieces as we go through this walkthrough.
This walkthrough is using example of Fast Food Menu, which contains information about menu items of fast food restaurant, organized in categories.
Pre-Requisites
VaultMap is developed for M-Files developers, that implement VAF or API applications that work with M-Files vault data. Most of the pre-requisites will be probably already installed, but here is the list:
- Visual Studio 2015 or higher
- M-Files Server 2015 or higher
- M-Files API (installed with M-Files, can be found in GAC)
- .NET Framework 4.5 or higher
1 Create M-Files vault
We will create very simple M-Files vault from scratch. Let's give it a name 'Fast Food Menu'.
If you want to skip creation part, you can download one of following vault examples:
IMPORTANT:
Keep in mind that aliases of M-Files entities are very important, because VaultMap is mapping objects according to set alias, which is considered to be unique for every M-Files entity.
1.1 Create two object types
Category
- Name (singular): Category
- Name (plural): Categories
- Aliases: OT.Category
- Name (singular): Menu Item
- Name (plural): Menu Items
- Aliases: OT.MenuItem
1.2 Update automatically created classes
- 'Category' class alias: CL.Category
- 'Menu Item' class alias: CL.MenuItem
1.3 Update automatically created property definition
Update automatically created property definition 'Category' as following:
- Name: Categories
- Alias: PD.Categories
1.4 Update aliases of built-in property definitions
- 'Name or title' property definition alias: PD.NameOrTitle
- 'Workflow' property definition alias: PD.Workflow
- 'State or title' property definition alias: PD.State
M-Files built in system properties can't be updated from M-Files Admin tool. It can be done only through API calls. We prepared simple windows application for doing this and you can donwload it from here.
1.5 Create new property definitions
Description
- Name: Description
- Data type: Text (multi-line)
- Content: Text
- Alias: PD.Description
- Name: Price
- Data type: Number (real)
- Alias: PD.Price
1.6 Add property definitions to classes
- Add property definition 'Description' to 'Category' class.
- Add property definitions 'Categories, Description, Price' to 'Menu Item' class.
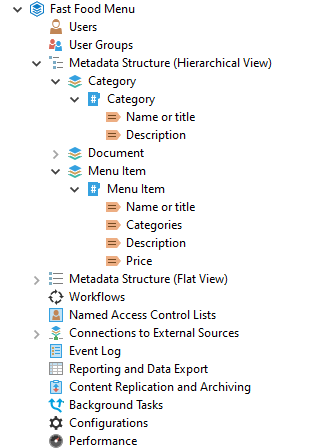
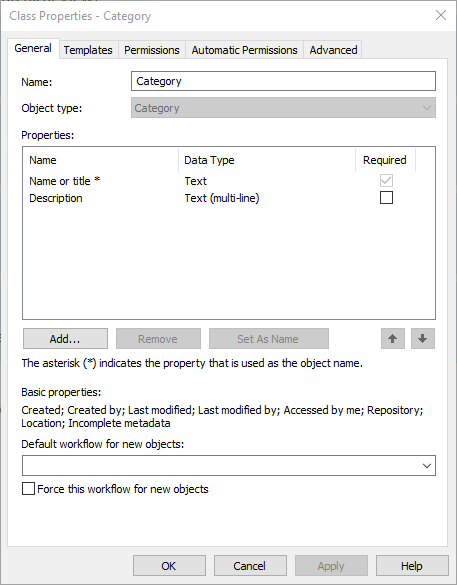
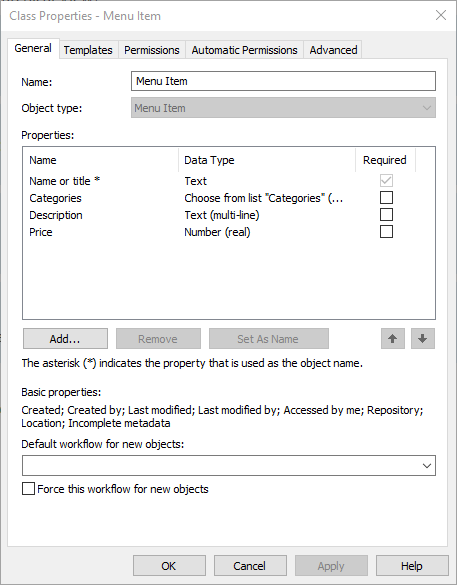
2 Create application
In this example we will make console application that will perform basic operations with created vault entities. You can download prepared solution here.
IMPORTANT:
If you decide to download demo project, have in mind that windows might block you some of the .dll and .exe files from package. In that case, you would need to find those files, open their property window and unblock them.
2.1 Create new solution
Create new solution and console application project with name "Fast Food Menu" in folder "D:\Fast Food Menu".
2.2 Prepare vault connection string
Prepare connection string for the vault that will be used later for building and accessing the vault. Connection string example can be following:
VaultGuid={EF3A707B-EE00-4F66-B3ED-00D9A877C70E};AuthType=3;UserName=username;Password=123456;Protocol=ncalrpc;UseObjectCaching=true
- VaultGuid - guid of the vault
- AuthType - authentication type for accessing the vault with provided credentials
- UserName - user name of login account with system administrator server role
- Password - password of the login account
- Protocol - protocol used for connection to the vault
- UseObjectCaching - flag if caching should be used for object values, which significantly improves performances
Keep in mind that m-files login used in connestion string should be also added to vault with permission of full control of vault.
2.3 Add references
- Add MFilesAPI as reference to the project.
- Install MFiles.VaultMap nuget package to the project.
2.4 Import VaultMap module
If you are going through this walkthrough step-by-step then this step can be skipped, because it is automatically performed immediately after installing MFiles.VaultMap nuget package. But if you are generating models at certain point after package installation, and if module is not found in "Package Manager Console", then it needs to be imported.
Get-VaultModels module can be found in Tools folder of installed VaultMap package, which in our case is folder "D:\Fast Food Menu\packages\MFiles.VaultMap.1.0.0\Tools" (folder might be different depending on project file location and installed version of package, which in our case is 1.0.0).
Importing module can be done in "Package Manager Console" window, by entering following line:
Import-Module '.\packages\MFiles.VaultMap.1.0.0\Tools\VaultMapModule.dll'
or in our case that would be also expressed as
Import-Module 'D:\Fast Food Menu\packages\MFiles.VaultMap.1.0.0\Tools\VaultMapModule.dll'
2.5 Generate vault entities
In this step we will generate files used by VaultMap. Because of adding new folders and files to the project, it is good to save/rebuild the project before this step is executed.
Make sure that in "Package Manager Console" window you have selected "Fast Food Menu" as your default project. Vault entities are now generated by executing following command:
Get-VaultModels -ConnectionString "VaultGuid={EF3A707B-EE00-4F66-B3ED-00D9A877C70E};AuthType=3;UserName=username;Password=123456;Protocol=ncalrpc;UseObjectCaching=true" -GenerateRepositories 1
- Get-VaultModels - method that generates entities
- ConnectionString "VaultGuid={EF3A707B-EE00-4F66-B3ED-00D9A877C70E};AuthType=3;UserName=username;Password=123456;Protocol=ncalrpc;UseObjectCaching=true" - connection string that we prepared in step 2.2
- GenerateRepositories 1 - flag if we want to generate suggested repositories, usually this is suggested for the first time, after that if we want to rebuild only models, this value can be set to 0
Note:
There is another way to execute Get-VaultModels without taking care what default project is selected. In that case we need to specify two more parameters - ProjectPath and ProjectName.
Get-VaultModels -ProjectPath "D:\Fast Food Menu\Fast Food Menu" -ProjectName "Fast Food Menu" -ConnectionString "VaultGuid={EF3A707B-EE00-4F66-B3ED-00D9A877C70E};AuthType=3;UserName=username;Password=123456;Protocol=ncalrpc;UseObjectCaching=true" -GenerateRepositories 1
- Get-VaultModels - method that generates entities
- ProjectPath "D:\Fast Food Menu\Fast Food Menu" - path of project for which we want to generate entities (location of .csproj file of the project)
- ProjectName "Fast Food Menu" - name of project and .csproj file
- ConnectionString "VaultGuid={EF3A707B-EE00-4F66-B3ED-00D9A877C70E};AuthType=3;UserName=username;Password=123456;Protocol=ncalrpc;UseObjectCaching=true" - connection string that we prepared in step 2.2
- GenerateRepositories 1 - flag if we want to generate suggested repositories, usually this is suggested for the first time, after that if we want to rebuild only models, this value can be set to 0
After execution of Get-VaultModels method is finished, Visual Studio will ask us to reload the project, and you should do so. There should be two new folders in project - VaultModels and VaultRepositories, containing generated VaultMap entities.
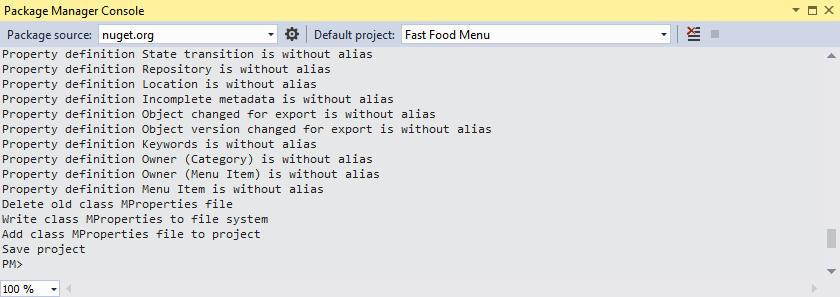
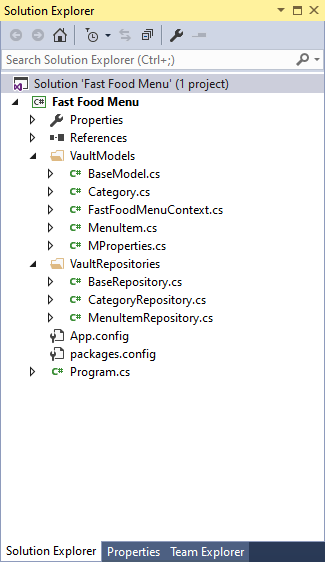
2.6 Update App.config
We should add connection string to the config file of project, in this case it is App.config.
Added config connection string would like as following:
- name - Name of connection string is important to be the same as name used in generated Context file, in our case it is "FastFoodMenuContext".
- connectionString - connection string that we prepared in step 2.2
At this moment we have our application ready for usage: we have built models and repositories, and we can access vault data.
2.7 Extending generated files
Generated models and repositories are built as partial classes, so they can be extended in different files. This might be useful if we want to extend our models and repositories with our custom made functions, and not to lose them after we decided to rebuild vault entities. Vault entities can be rebuilt if we have changes in vault structure.
In our example we will extend MenuItemRepository with two new methods that will be used later in code.
Create new folder "RepositoryPartials" in project root. Create new file "MenuItemRepository.cs" under created folder.
Add following code to the newly create file:
2.8 Building services to process data
We will build now two separate service files in order to process data from the vault.
In these methods we will generate repository instances and read and write data of the vault.
In root of the project add two following files with code:
- CategoryService.cs
- MenuItemService.cs
2.9 Implement calls of services
In final step we are adjusting Program.cs file of the project, where we should display 'main menu' of the application, and call service methods based on user input.
Update Program.cs file as following:
Solution structure should look like following:
At this moment we have application ready for usage. After running the application we can read/create/update/delete data from the M-Files vault.
3 Further improvements
M-Files VaultMap is at its own very beginning. We are aware of fact that it can be still improved a lot, and we ensure you that we are going in that direction. We are building it step by step, and your support is very important for us, so we would like to hear from you about all the ideas for further improvements.
Here is list of some of them that we have in our mind:
-
Creating object instances of lookup properties - in our example this means that instead of having "List
Categories" property in "MenuItem" class, we have "List Categories" property. - Workflow mapping - similar to previous improvement, we would have object for workflows and states
- Value lists mapping
- Permissions handling
- NVS handling
- Better installation process - currently module methods for generating entities is too complex, we should build better and more simple way of executing that method
- Partial mapping of entities - there should be way to select entities we want to map to objects
4 Contact us
We would like to hear your comments, no matter if they are good or not.
Write us on: dejan.efremov@outlook.com